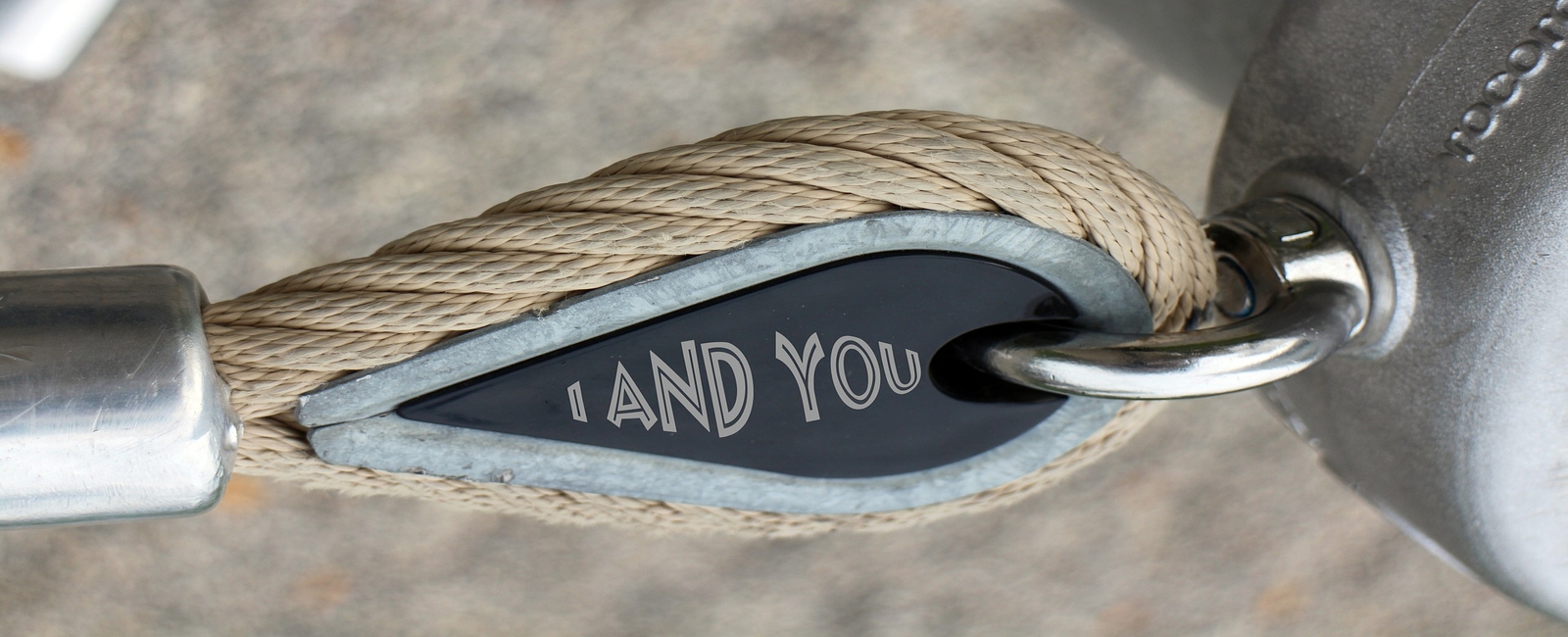
I will explain how to create a TextView with inside a clickable link in Android.
Do you want to open a static link without doing other operations? So just follow the first part of this tutorial! Otherwise, if you want to have more control on the link customization and operations after the user taps on it, follow the second part of the tutorial.
Let’s create an example TextView in your layout like this one:
<TextViewandroid:id="@+id/messageWithLinkTextView"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_centerInParent="true"android:gravity="center"android:text="@string/messageWithLink" />
Create this string in strings.xml
:
<string name="messageWithLink">This is a message with a link inside!\n<a href="https://www.google.com/">Tap here to open it!</a></string>
Write this code in your activity/fragment:
@Bind(R.id.messageWithLinkTextView)TextView messageWithLinkTextView;@Overridepublic void onViewCreated(View view, Bundle savedInstanceState) {super.onViewCreated(view, savedInstanceState);ButterKnife.bind(this, view);messageWithLinkTextView.setMovementMethod(LinkMovementMethod.getInstance());}
Please note: I’m using ButterKnife library to bind fields and views (I suggest you to have a look at this fantastic library!)
You are done! You should get a TextView like the one in the following screenshot and when you tap on the hyperlink the browser should open! Cool!
P.S. unfortunately I didn’t find a way of achieving this only using XML properties. If anyone knows how to do it, please let me know in the comments!
Create another TextView in your layout:
<TextViewandroid:id="@+id/messageWithSpannableLinkTextView"android:layout_width="wrap_content"android:layout_height="wrap_content"android:layout_centerInParent="true"android:gravity="center"tools:text="@string/messageWithSpannableLink" />
Create another string resource in strings.xml
:
<string name="messageWithSpannableLink">This is a message with a spannable link inside!\nTap here to open it!</string>
Please note: this time there is no tag inside the string!
Now write this code in your activity/fragment:
@Bind(R.id.messageWithSpannableLinkTextView)TextView messageWithSpannableLinkTextView;@Overridepublic void onViewCreated(View view, Bundle savedInstanceState) {super.onViewCreated(view, savedInstanceState);ButterKnife.bind(this, view);SpannableString spannableString = new SpannableString(getString(R.string.messageWithSpannableLink));ClickableSpan clickableSpan = new ClickableSpan() {@Overridepublic void onClick(View textView) {startActivity(new Intent(Intent.ACTION_VIEW, Uri.parse("https://www.google.com")));}};spannableString.setSpan(clickableSpan, spannableString.length() - 20, spannableString.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);messageWithSpannableLinkTextView.setText(spannableString, TextView.BufferType.SPANNABLE);messageWithSpannableLinkTextView.setMovementMethod(LinkMovementMethod.getInstance());}
You are done! You should get a TextView like the one in the following screenshot and when you tap on the hyperlink the ClickableSpan->onClick() method should be called, so you can do your operations and let the browser open the link! Cool!
Check out the complete source code on my GitHub here!